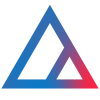 |
Diarkis C++Client Library API Document
|
Go to the documentation of this file.
5 #include <condition_variable>
22 #include "logging/LoggerFactory.h"
25 #define ENABLE_LOGGER 1
108 static bool DiarkisInit(
const char* logDirName,
LogOutType out =
DEBUG_OUT,
bool bLog =
true, std::shared_ptr<ILoggerBackend> customLogger =
nullptr);
116 static bool DiarkisInit(
const std::string& logDirName,
119 std::shared_ptr<ILoggerBackend> customLogger =
nullptr);
127 static bool DiarkisDestroy();
135 void UpdateComponents();
143 bool ConnectTcp(
void);
151 virtual bool SetupTcp(
void);
161 bool ConnectTcp(
const char* tcpEndpoint);
169 bool ConnectTcp(
const std::string& tcpEndpoint);
177 bool ConnectUdp(
void);
197 bool GetEndpoint(
const char* host,
const char* clientKey,
const char* serverType,
char* endpoint,
size_t endpointBuf);
205 bool GetEndpoint(
const std::string& host,
const std::string& clientKey,
const std::string& type,
Diarkis::StdString& endpoint);
213 virtual bool SetupUdp(
void);
227 bool ConnectUdp(
const char* udpEndpoint,
const char* type,
struct AuthInfo* auth);
235 bool ConnectUdp(
const char* udpEndpoint);
243 bool ConnectUdp(
const std::string& udpEndpoint);
263 bool ConnectUdpAsync(
const char* udpEndpoint);
271 bool ConnectUdpAsync(
const std::string& udpEndpoint);
285 bool ConnectUdpDualModeAsync(
const char* udpEndpoint);
293 bool ConnectUdpDualModeAsync(
const std::string& udpEndpoint);
303 void GetAuthInfo(
struct AuthInfo* auth);
313 virtual void SetupP2P(
void);
331 virtual void SetupRoom(
bool bRetry =
false);
345 virtual void SetupSession(
bool bRetry =
false);
353 virtual void SetupRpc();
363 virtual void SetupField(
void);
377 virtual void SetupGroup(
bool bRetry);
387 virtual void SetupMatchMaker(
void);
397 virtual void SetupDirectMessage(
void);
414 virtual void SetupProfile(uint32_t interval, uint32_t buffer,
bool p2pRandomStart =
true);
422 void SetHost(
const char* host);
430 void SetHost(
const std::string& host);
454 void SetClientKey(
const char* clientKey);
462 void SetClientKey(
const std::string& clientKey);
486 void SetSid(
const char* sid);
494 void SetSid(
const std::string& sid);
502 std::shared_ptr<LoggerFactory> GetLoggerFactory();
510 std::shared_ptr<ILoggerBackend> GetConsoleLoggerBackend();
518 std::shared_ptr<ILoggerBackend> GetDebugLoggerBackend();
526 std::shared_ptr<DiarkisUdpBase> GetUdpBase();
534 std::shared_ptr<DiarkisTcpBase> GetTcpBase();
542 std::shared_ptr<DiarkisP2PBase> GetP2PBase();
550 std::shared_ptr<DiarkisRoomBase> GetRoomBase();
558 std::shared_ptr<DiarkisSessionBase> GetSessionBase();
566 std::shared_ptr<DiarkisGroupBase> GetGroupBase();
574 std::shared_ptr<DiarkisFieldBase> GetFieldBase();
582 std::shared_ptr<DiarkisMatchMakerBase> GetMatchMakerBase();
590 std::shared_ptr<DiarkisRpcBase> GetRpcBase();
598 std::shared_ptr<DiarkisDirectMessageBase> GetDirectMessageBase();
606 std::shared_ptr<Diarkis::Network::IDiarkisProfile> GetProfile();
649 bool IsOffline(
void);
668 uint16_t GetP2PConnectedNum(
void);
701 void CreateRoom(uint16_t maxMembers,
bool allowEmpty,
bool join, uint16_t ttl, uint32_t interval);
731 void RandomJoinRoom(uint16_t maxMembers, uint16_t ttl, uint32_t interval,
bool allowEmpty);
761 void JoinRoom(
const char* roomID);
769 void JoinRoom(
const std::string& roomID);
789 void SendMessageToRoom(
const char** memberIDs,
size_t memberIDsCount,
const uint8_t* payload,
size_t payloadSize,
bool reliable);
797 void SendMessageToRoom(
const std::vector<std::string>& memberIDs,
const std::vector<uint8_t>& payload,
bool reliable);
811 void SendLeaveRoom();
827 void FindByTypeRoom(uint32_t roomType, uint32_t limit);
843 void RegisterRoom(uint32_t roomType,
const char* roomName,
const char* roomMetadata);
851 void RegisterRoom(uint32_t roomType,
const std::string& roomName,
const std::string& roomMetadata);
867 void SendMigrateRoom(
void);
877 void SendGetOwnerID(
void);
887 void SendGetMemberIDs(
void);
922 void SendCreateGroup(
bool allowEmpty,
bool join, uint16_t ttl);
940 void SendJoinGroup(
const char* groupID,
const char* message);
948 void SendJoinGroup(
const std::string& groupID,
const std::string& message);
968 void SendRandomJoinGroup(uint16_t ttl,
const char* message, uint32_t interval = 200);
976 void SendRandomJoinGroup(uint16_t ttl,
const std::string& message, uint32_t interval = 200);
990 void SendLeaveGroup(
const char* groupID,
const char* message);
998 void SendLeaveGroup(
const std::string& groupID,
const std::string& message);
1014 void ClearUdpBuffer();
1048 bool RequestEndpointAsync(
const char* host,
1049 const char* clientKey,
1053 uint32_t affinityMask,
1054 uint32_t stackSize);
1062 bool RequestEndpointAsync(
const std::string& host,
1063 const std::string& clientKey,
1064 const std::string& type,
1067 uint32_t affinityMask,
1068 uint32_t stackSize);
1078 AsyncGetEndpointStatus GetEndpointAsyncStatus()
const;
1099 void InitAndStartup(
bool bInitBaseClass);
1107 static void CreateLogFolder();
1123 void SchedulerAddNotifyEvent();
1131 static void InitializeLoggerEnvironment();
1139 static bool IsLogOutputTypeFile();
1228 void Update(
bool forceUpdate);
1269 void TerminateGetEndpointAsyncThread();
1349 std::shared_ptr<DiarkisDirectMessageBase>
dmBase_;
1357 std::shared_ptr<Diarkis::Network::IDiarkisProfile>
profile_;
1413 static std::shared_ptr<Diarkis::StdString>
logDir_;
AsyncGetEndpointStatus
Running status of the process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:58
std::shared_ptr< Diarkis::System::DiarkisThread > getEndpointAsyncThread_
The thread used by the process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:1570
std::shared_ptr< DiarkisTcpBase > tcpBase_
Pointer to DiarkisTcpBase class.
Definition: DiarkisInterfaceBase.h:1277
@ DEBUG_AND_FILE_OUT
Definition: DiarkisInterfaceBase.h:39
AuthInfo authInfo_
Authentication information.
Definition: DiarkisInterfaceBase.h:1405
std::shared_ptr< DiarkisP2PBase > p2pBase_
Pointer to DiarkisP2PBase class.
Definition: DiarkisInterfaceBase.h:1293
Diarkis::StdUniquePtr< Diarkis::StdVector< DiarkisInterfaceBase * > > instances_
A list of the instances that are currently executing the update process.
Definition: DiarkisInterfaceBase.h:1244
std::shared_ptr< DiarkisRoomBase > roomBase_
Pointer to DiarkisRoomBase class.
Definition: DiarkisInterfaceBase.h:1301
std::shared_ptr< DiarkisRpcBase > rpcBase_
Pointer to DiarkisRpcBase class.
Definition: DiarkisInterfaceBase.h:1341
static std::shared_ptr< ILoggerBackend > aggregatedLoggerBackend_
A logger interface that is used in the LoggerFactory.
Definition: DiarkisInterfaceBase.h:1477
AsyncGetEndpointStatus asyncGetEndpointStatus_
The progress of the process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:1538
@ CONSOLE_OUT
Definition: DiarkisInterfaceBase.h:38
Diarkis::StdString host_
Address of the HTTP server to connect to.
Definition: DiarkisInterfaceBase.h:1365
Diarkis::StdString sid_
Session ID.
Definition: DiarkisInterfaceBase.h:1397
Diarkis::StdString serverType
Definition: DiarkisInterfaceBase.h:1505
std::mutex mutex_
A mutex object for the list.
Definition: DiarkisInterfaceBase.h:1236
std::shared_ptr< DiarkisFieldBase > fieldBase_
Pointer to DiarkisFieldBase class.
Definition: DiarkisInterfaceBase.h:1325
static std::shared_ptr< Diarkis::StdString > logDir_
Log output directory name.
Definition: DiarkisInterfaceBase.h:1413
Definition: DiarkisInterfaceBase.h:1202
std::shared_ptr< LoggerFactory > loggerFactory_
Pointer to LoggerFactory.
Definition: DiarkisInterfaceBase.h:1429
AuthInfo asyncAuthResult_
The result of the process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:1562
std::atomic< bool > isRunning_
Runtime loop exit flag.
Definition: DiarkisInterfaceBase.h:1159
Diarkis::StdString clientKey
Definition: DiarkisInterfaceBase.h:1504
Contains information about a specific result value. Can be compared against another Result structure....
Definition: result.h:37
std::unique_ptr< T, Diarkis::DiarkisAllocatorDeleter< T > > StdUniquePtr
Definition: common.h:384
std::shared_ptr< DiarkisMatchMakerBase > matchMakerBase_
Pointer to DiarkisMatchMakerBase class.
Definition: DiarkisInterfaceBase.h:1333
static ActiveInstanceList activeInstances_
A list of active instances.
Definition: DiarkisInterfaceBase.h:1253
std::shared_ptr< DiarkisSessionBase > sessionBase_
Pointer to DiarkisSessionBase class.
Definition: DiarkisInterfaceBase.h:1309
const Diarkis::StdString uid_
My user ID.
Definition: DiarkisInterfaceBase.h:1381
std::shared_ptr< IDiarkisLogger > logger_
Pointer to logger class.
Definition: DiarkisInterfaceBase.h:1421
static std::shared_ptr< ILoggerBackend > fileLoggerBackend_
Logger for file output.
Definition: DiarkisInterfaceBase.h:1461
@ FILE_OUT
Definition: DiarkisInterfaceBase.h:36
bool noWait_
Whether it need to enter the wait by using the condition variable.
Definition: DiarkisInterfaceBase.h:1191
std::condition_variable sync_condition_
A condition_variable that will be used for controlling the process launch timing.
Definition: DiarkisInterfaceBase.h:1183
static std::shared_ptr< LoggerFactory > globalLoggerFactory_
A LoggerFactory that will be used for logging of the global process of the DiarkisInterfaceBase.
Definition: DiarkisInterfaceBase.h:1261
Diarkis::StdString serverType_
Server type.
Definition: DiarkisInterfaceBase.h:1389
Diarkis::StdString asyncEndpointResult_
The result of the process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:1554
@ DEBUG_OUT
Definition: DiarkisInterfaceBase.h:35
AsyncGetEndpointArgs asyncGetEndpointArgs_
The arguments passing to the asynchronous getting the endpoint process.
Definition: DiarkisInterfaceBase.h:1530
std::shared_ptr< Diarkis::Network::IDiarkisProfile > profile_
Pointer to IDiarkisProfile class.
Definition: DiarkisInterfaceBase.h:1357
static LogOutType logOutType_
Type of debug output.
Definition: DiarkisInterfaceBase.h:1485
std::mutex connectioninfoMutex_
The mutex for the variables that are modified in the GetEndpoint method.
Definition: DiarkisInterfaceBase.h:1514
Diarkis::StdString host
Definition: DiarkisInterfaceBase.h:1503
LogOutType
Type of log output.
Definition: DiarkisInterfaceBase.h:33
std::atomic< bool > internalSchedulerUpdated_
A flag enabled if the scheduler is updated.
Definition: DiarkisInterfaceBase.h:1586
@ FILE_OUT_TO_SPECIFIC_PATH
Definition: DiarkisInterfaceBase.h:37
static bool bOutputLog_
Whether to log output or not.
Definition: DiarkisInterfaceBase.h:1493
Base class for interfaces that manipulate the Diarkis library.
Definition: DiarkisInterfaceBase.h:49
static std::shared_ptr< ThreadedLoggerBackend > threadLoggerBackend_
Threaded loggers for collective output.
Definition: DiarkisInterfaceBase.h:1437
Diarkis::StdString clientKey_
Client key of the HTTP server to connect to.
Definition: DiarkisInterfaceBase.h:1373
@ CUSTOM
Definition: DiarkisInterfaceBase.h:40
std::shared_ptr< DiarkisDirectMessageBase > dmBase_
Pointer to DiarkisDirectMessageBase class.
Definition: DiarkisInterfaceBase.h:1349
DiarkisThreadPolicy
Thread scheduling policy.
Definition: Thread.h:44
std::shared_ptr< Diarkis::System::DiarkisThread > runtimeThread_
Runtime loop thread.
Definition: DiarkisInterfaceBase.h:1167
RuntimeThreadData()
Definition: DiarkisInterfaceBase.h:1143
std::mutex asyncGetEndpointMutex_
The mutex for the whole process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:1522
std::shared_ptr< DiarkisUdpBase > udpBase_
Pointer to DiarkisUdpBase class.
Definition: DiarkisInterfaceBase.h:1285
bool asyncGetEndpointResult_
The result of the process of getting the endpoint asynchronously.
Definition: DiarkisInterfaceBase.h:1546
static std::shared_ptr< ILoggerBackend > customLoggerBackend_
Custom logger.
Definition: DiarkisInterfaceBase.h:1469
std::shared_ptr< DiarkisGroupBase > groupBase_
Pointer to DiarkisGroupBase class.
Definition: DiarkisInterfaceBase.h:1317
static std::shared_ptr< ILoggerBackend > debugLoggerBackend_
Logger for debug output.
Definition: DiarkisInterfaceBase.h:1453
#define DIARKIS_API
Definition: common.h:47
The arguments passing to the asynchronous getting the endpoint process.
Definition: DiarkisInterfaceBase.h:1501
static RuntimeThreadData runtimeThreadData_
A data of the runtime thread.
Definition: DiarkisInterfaceBase.h:1200
std::mutex sync_mutex_
A mutex object that will be used by the condition_variable.
Definition: DiarkisInterfaceBase.h:1175
Definition: DiarkisInterfaceBase.h:1141
static std::shared_ptr< ILoggerBackend > consoleLoggerBackend_
Logger for console output.
Definition: DiarkisInterfaceBase.h:1445